Hier findest Du die wesentlichen Dinge, die Du brauchst um ein TableView zu durchsuchen. Zuerst ziehst Du mal eine Searchbar aus dem Objektkatalog und erstellst im Code ein Outlet.
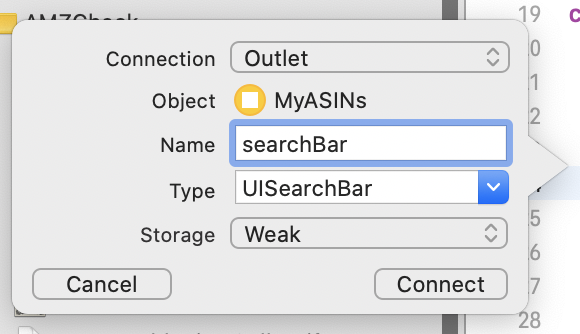
Nachdem Du das Outlet im Quelltext gesetzt hast, setze das delegate.
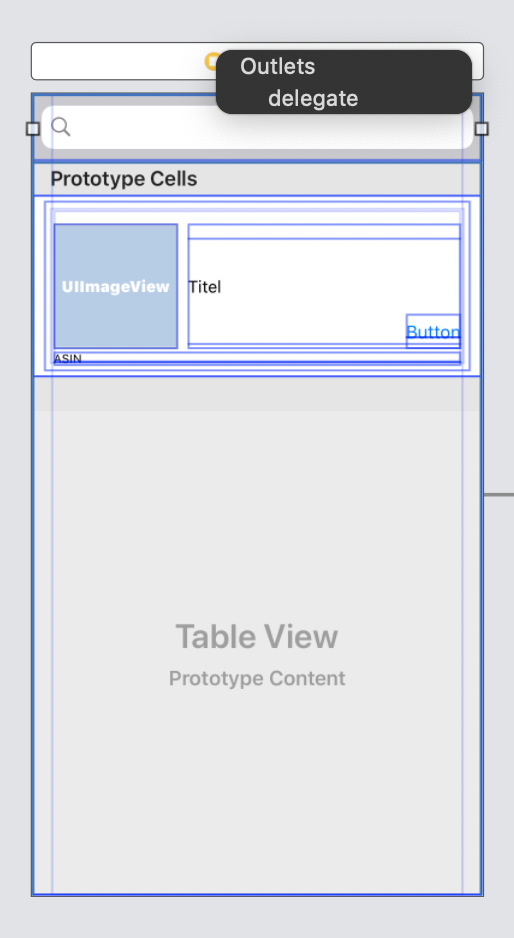
Jetzt benötigen wir noch eine Variable „searching“ sowie ein weiteres 2-dimensionales Array. Die Variable searching bekommt den Wert true, während eine Suche stattfindet. Hier im Beispiel siehst du die Variable für das 2. Array, dass baugleich mit dem Array results ist. In das 2. Array wird jeweils das Filterergebnis gesetzt. Jetzt weißt Du, warum wir die Boolean Variable „searching“ benötigen. So kann man für alle weiteren Aktionen im Code abfragen, aus welchem Array man sich gerne bedienen möchte. Je nachdem ob halt gerade gesucht wird oder die Komplette Liste im Tableview dargestellt wird.
var searching = false var filteredresults: [[String: String]]? = [[String: String]]()Nun diese extension hinzufügen. Hier wird das 2. Array filteredresults mit dem Filterergebnis gefüllt und festgelegt ob unsere Variable searching true oder false gesetzt wird. Logischerweise wird
seraching = falsegesetzt, wenn
filteredresults!.count == 0Datensätze enthält.
extension ViewController: UISearchBarDelegate { func searchBar(_ searchBar: UISearchBar, textDidChange searchText: String) { filteredresults = self.results!.filter ({ $0["ASIN"]!.localizedCaseInsensitiveContains(searchText) }) if(filteredresults!.count == 0){ searching = false }else{ searching = true } self.tableView.reloadData() } }
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { if (searching){ return filteredresults!.count }else { return (results!.count) } }
Beim füllen der Felder in der Tableview Cell darauf achten, daß auch hier aus dem richtigen Array abgegriffen wird.
if (searching){ cell.lblASIN.text = filteredresults![indexPath.row]["ASIN"] } else { cell.lblASIN.text = results![indexPath.row]["ASIN"] }
Hier solltest Du natürlich auch darauf achten, dass numberOfRowsInSection jeh nach Suchzustand korrekt zurückgeliefert wird.
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { if (searching){ return filteredresults!.count }else { return (results!.count) } }